What I figured out just by watching the pre-intro – they are more low-key about the event than usual. No wild build-up to the keynote, music is relaxing and downtempo. And they are very blue
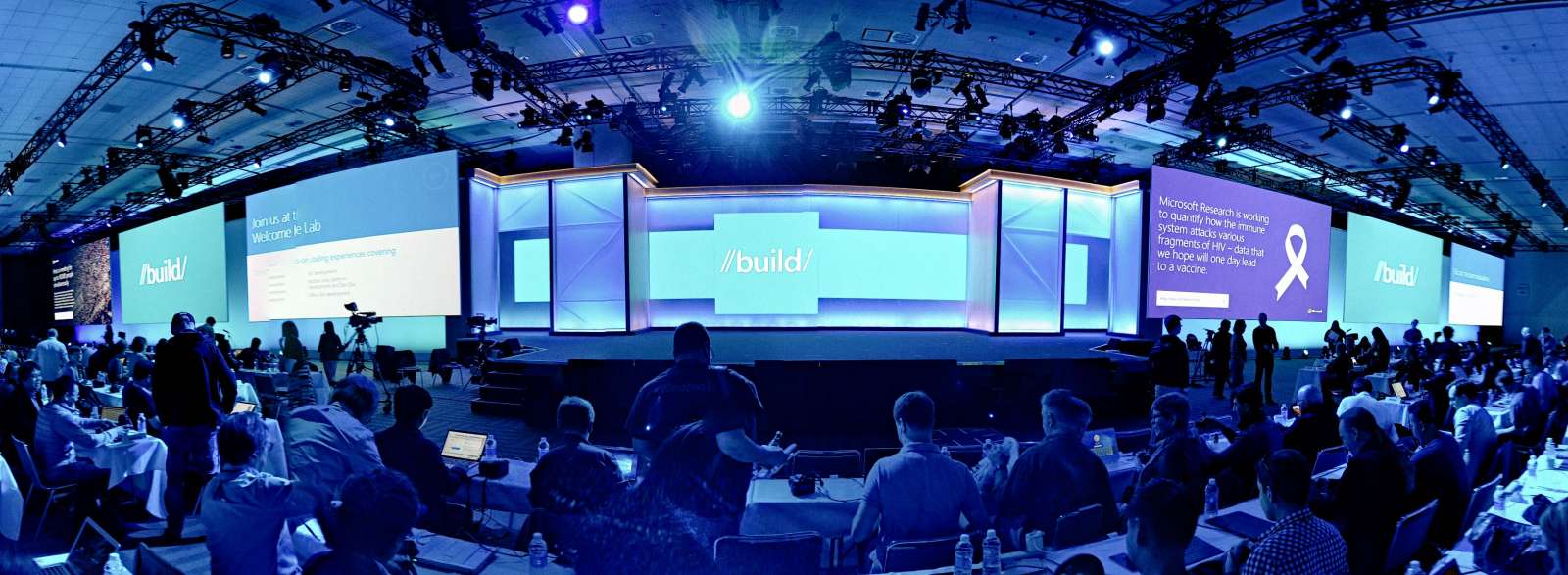
Satya Nadella started by taking over the stage. Taking over – he is full of energy, everyone loves him (what is not to love on the guy who brought you SQL on Linux, Office for iPad, etc.) He quickly points out how dev conference is a dev conference: it's about networking and being inspired. This unique characteristic what he wants to build on and celebrate. He brought up topics in his opening speech that focused on society and how it can be a mainstream thing; how it can either hurt or help it; how it should empower you. He also was overly optimistic, and said, that optimism must be buoyed by making the right choices. And of course, the mantra of 'mobile first, cloud first'. "Cloud is not a single destination. It's a new form of computing" he said.
 Creating, building and reinventing is the 3 main pillars for cloud and is Microsoft's ambitions in this space. Windows 10 is off to a great start – he said. New platform for human voice, fingerprint, and more? "It's such a great time to be a Windows Developer." And there are 270 million Windows 10 installation (5 billion store visits); if we look at the 1 billion installation in 3 years, we are not that far from the forecast – and actually is the fastest ever adoption.
Terry Myerson starts with "Hello Developers" – anyone wants to continue with "Developers! Developers!" and large amount of sweat? Windows 10 anniversary update would still come free.It contains new ink experience (with ink workspaces for last used ink apps), biometrics for Microsoft Edge (got demo of it, using biometric scanner to log into the website), support for Windows 10 on XBOX, enabling general XBOX One development (e.g. any retail XBOX One can be used as a dev kit/machine, using the same UWP username/account). You get nice controller support for selection, focus, etc. But also you get speech recognition and more on the XBOX, and with full VS.NET debugging experience. Hololens gets also refresh during the summer. Bryan Roper stayed in character with his clothes and style, no surprise They are bringing pen & paper even further into the focus. He demoed the new ink features – front and center; and he just started! He was able to write with ink and that got contextive focus from Cortana providing full integration for sticky notes ('Call mom tomorrow' gets translated into reminder). Can I get an 'Ohhh' – Ohhh!
We saw demo of a virtual ruler using whiteboard/sketchboard as well, and just tweeted it from the application. Important to know how you get something new – ink and touch together, enables for example amazing features in PhotoShop. Also, we got demo of 3D maps and ink usage – points of interest, plotted routes, etc. Also we got demo of using ink in Office – Word to remove texts, for highlighting; Powerpoint to show how items can be lined up, etc. Generally Microsoft is creating a layer of contextual computer learning to make the stylus smart and useful in a way you want it to be. It's more of a real digital pen than ever.
As part of the keynote, Facebook was announcing that it's bringing it's ad network to Windows 10 universal apps, that will considerably help monetizing applications.
And at last (35 minutes in) "It's about time we looked at some code!" Kevin Gallo is on stage! "We want Windows to be home for Developers" Part of UWP got AnimationFX platform introduced with hovers, blurring, flowing, shadows. All GPU accelerated! And also with deep ink integration. Here comes "Hello world" for ink, with InkCanvas and InkToolbar. The new design language seems to merge Material, Jellybean and existing metro; flat & robotic, but layering and animations.
Visual Studio 2015 Update 2 and Anniversary SDK preview was announced, that enables use the features above. Next topics was web apps, e.g. how to make a desktop application using only web technologies. And yes, BASH shell is coming to Windows. Not VM, not cross compiled. New Linux subsystem supports this. This enables people stay in BASH, emacs, etc., e.g. with their known tools but still use Windows.
And officially announced was Centennial – Win32 app converter, to continue running your desktop application; they will get built into WISE, Installshield, etc. And with doubleclick support for .appx, with support for accessing live tiles, toasts, etc. You only need to add the extra functionality as a separate DLL and load that on demand when you are. This will help move the 16.000.000+ applications to the store.
 And the expected demo from Xamarin – nice addition was a remote XLB editor from Visual Studio next to rest of nice, immersive features. Ok, Terry Myerson just said Bash and DOS in the same sentence. If you are doing a drinking game, this is the time to drink the whole bottle. They just mentioned we will focus on gaming now – Phil Spencer first, Kipman on Hololens next. Phil will surely have a hard time convincing people that UWP is the best platform for writing games. Killer Instinct, Quantum Break, Tomb Raider, Gears of War, etc all being UWP. In May they add GSync and FreeSync instead of VSync. DX12 is coming full speed, for full screen games, with overlays, and more. They actually demoed Age of Empires (directly from steam!), Witcher 3, running as an UWP, full frame rate, full screen.
This ins't a hobby. It's a commitment – said Microsoft about Xbox One Universal apps. and the preview of dev mode is available from today. And DX12, which makes it all possible is the fastest adoption of API so far.

And yes, shipping for Hololens is happening today. And they are opensourcing Galaxy Explorer. We got a good demo on how medical students can use Hololens. "To think and see beyond the possible" Also demo of Destination Mars, in Kennedy Space center and onto the Build conference. At last people can try doing everything Matt Damon did in Martian was wrong. Hololens do transform your world.
And Nadella is back to 'switch gears'. We will help computers learn so that they can help in everyday tasks. It's not about man versus machines. It's about man with machines! By augmenting human abilities and with experiences, being inclusive and with earning trust. Human language is the new UI. Bots are the new apps, digital assistants are meta apps. Intelligence gets infused into all interactions. As a result of this idea, Cortana is building to be an actual assistant, not an assistant that's limited to platform/device. Right now Cortana answers one million spoken questions per day, 1000+ application connected. New version of Cortana will work on lock screen, having a full screen experience; also having an integration with Outlook. "Sent Chuck to powerpoint I worked on yesterday"; by sending text message it automatically sets up meeting and moves other meetings around. Also will figure out it's during lunch time, so it will try to suggest eating out; all based on the connected applications. This happens based on a connected application which looks into calendar and decides to toast based on that. Based on receipt in email, Cortana suggested submitting expenses. "what toy store did I got to last year at build?" Cortana Developer Preview invites are available!
Growing number of conversation canvases are there – slack, kik, groupme, wechat, line, skype, email, sms, etc. They look into making these into rich conversational canvases. Where does this lead? Into working Cortana into the applications via using bots. Like transcribing video messages, having rich bing powered cards, brokering conversation with 3rd parties, knowing context from previous conversations, and sharing context between brokers. Like not only booking calendar, but booking hotel, suggesting to contact people, etc., this working for typed text, voice but also for realtime video and for hololens. Cortana really behaves like a real assistant – though making available the newly announced skype bot sdk; with a hackathon to kick it off; and is available in the applications from today; based on Microsoft Bot Framework (with bot builder sdk for nodejs and C# available from github), Cognitive Services (new website with 22 services at start at https://www.microsoft.com/cognitive-services, and with a demo ofhttp://captionbot.ai/ and CRIS recognition of voice) and Machine Learning (with many tools to teach the system on the fly and involve humans to help when machine is stuck). The idea is to have cognitive micro-services that you could use from each of the conversation canvases, not only from Skype. Also a very nice (actually, I'd say, amazing) demo for people for disabilities on how to use these services in action.
The summary of the day: Empower developers!
|